Solidityにおけるstruct(構造体)の書き方について解説します。
構文
Solidityにおけるstruct(構造体)の書き方ですが、以下のようにします。
構造体の中身ですが、データ型と変数名をセットにして変数を定義します。
struct 構造体名 {
データ型1 変数名1;
データ型2 変数名2;
データ型n 変数名n;
}
サンプルコード
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
contract Test {
// 構造体
struct User {
string name;
uint age;
address wallet;
}
User[] public users;
function addUser(string memory _name, uint _age, address _wallet) public {
User memory newUser = User({
name: _name,
age: _age,
wallet: _wallet
});
users.push(newUser);
}
function getUser(uint _index) public view returns (string memory, uint, address) {
User memory user = users[_index];
return (user.name, user.age, user.wallet);
}
}
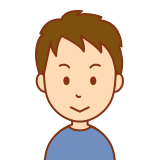
struct(構造体)は複数の変数をひとまとめにして扱いたいときに使用します。サンプルコードでは、ユーザーごとの名前/年齢/ウォレットアドレスを管理したいため、変数を個別に定義せず、Userという構造体により一つの変数として扱うようにしています。