This section explains how to compare strings in Solidity.
Syntax
To compare strings in Solidity, do the following.
keccak256(abi.encodePacked(string1)) == keccak256(abi.encodePacked(string2))
It is also possible to use string byte-string conversion (bytes()) instead of ABI encoding (abi.encodePacked()).
keccak256(bytes(string1)) == keccak256(bytes(string2))
Either method is acceptable, but the latter is likely to be only slightly less costly (gas).
Sample Code
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
/* Method using ABI encoding (abi.encodePacked()) */
function compareStringsByAbiEncoding(string memory s1, string memory s2) public pure returns(bool){
// compare strings
return keccak256(abi.encodePacked(s1)) == keccak256(abi.encodePacked(s2));
}
/* Method using string byte-string conversion (bytes()) */
function compareStringsByBytes(string memory s1, string memory s2) public pure returns(bool){
// compare strings
return keccak256(bytes(s1)) == keccak256(bytes(s2));
}
}
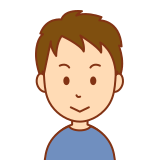
Because Solidity cannot handle strings as they are due to EVM (Ethereum Virtual Machine), it is necessary to encode strings into byte strings with abi.encodePacked() or bytes() or to hash strings with the keccak256 function. Solidity is different from other programming languages and can be confusing, but you will gradually get used to it.