This section explains how to get the number of characters (string length) in Solidity.
Syntax
To get the number of characters in Solidity, do the following.
bytes(string).length
Since the length method cannot be used on a string as is, the string must first be converted to a byte string with the bytes function.
Also note that for non-English characters such as Japanese and Chinese, one character is calculated as 3, 4 bytes (3, 4 characters) because it is a multibyte character.
Sample Code
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
function getStrLen(string memory str) public pure returns(uint) {
// Get the number of characters (string length)
// * Note that for non-English characters, 1 character is calculated as 3, 4 bytes (3, 4 characters)
return bytes(str).length;
}
}
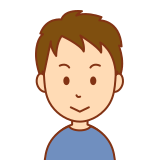
In Solidity, it is difficult to handle non-English characters (multibyte characters). You can implement it on your own, or you can use external libraries such as solidity-stringutils.