This section explains how to write if statements (conditional branching) in Solidity.
Syntax
There are three patterns of if statements.
- if statement only (single condition)
- if - else (other condition)
- if - else if (other specific conditions)
Each is explained below.
A summary table is also provided for comparison operators (inequality signs used for conditional judgments).
if statement only (single condition)
The syntax for if statement only (single condition) is as follows.
// if the condition is true
if (condition) {
Processing
}
if - else (other condition)
The syntax for if - else (other condition) is as follows.
// if the condition is true
if (condition) {
Processing
// otherwise
} else {
Processing
}
if - else if (other specific conditions)
The syntax for if - else if (other specific conditions) is as follows.
The “else if” clause can be repeated any number of times.
// if the condition is true
if (condition) {
Processing
// otherwise (if the condition is true)
} else if (condition) {
Processing
// otherwise (if the condition is true)
} else if (condition) {
Processing
// otherwise
} else {
Processing
}
Comparison Operators (inequality signs used for conditional judgments)
A table summarizing comparison operators is provided below.
Comparison Operator | Meaning |
---|---|
== | Equal |
!= | Not equal |
< | Less than |
> | Greater than |
<= | Less than or Equal to |
>= | Greater than or Equal to |
Sample Code
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
/* if statement only (single condition) */
function checkAgeIf(uint8 age) public pure returns(string memory) {
string memory message = "";
// Under 18
if (age < 18) {
message = "Minors.";
}
return message;
}
/* if - else (other condition) */
function checkAgeIfElse(uint8 age) public pure returns(string memory) {
string memory message = "";
// Under 18
if (age < 18) {
message = "Minors.";
// Otherwise
} else {
message = "Adults.";
}
return message;
}
/* if - else if (other specific conditions) */
function checkAgeIfElseIf(uint8 age) public pure returns(string memory) {
string memory message = "";
// Under 18
if (age < 18) {
message = "Minors.";
// Under 20
} else if (age < 20) {
message = "Adults. Enjoy your last teenage years!";
// Under 30
} else if (age < 30) {
message = "Adults. You are still in your twenties, young, young!";
// Under 40
} else if (age < 40) {
message = "Adults. Your 30s will pass in the blink of an eye!";
// Otherwise
} else {
message = "Adults. Let's stay healthy!";
}
return message;
}
}
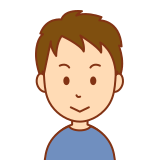
The if statement is essential knowledge for writing code! Be sure to keep it in mind. Conditional operators such as “or” and “and” are explained in a separate article. Please refer to it as well if you like.