This section explains how to initialize the array in Solidity.
Syntax
There are two types of arrays: “Fixed-Length Array” and “Variable-Length Array (Dynamic Array)”.
The syntax for each is described below.
Fixed-Length Array
As the name suggests, a Fixed-Length Array is “an array with a fixed number of elements (array length)”.
Fixed-Length Array can be initialized in either of the following ways.
- Initialize at the same time as the array declaration
- Declare the array first and initialize it later
Initialize at the same time as the array declaration
When initializing an array at the same time as its declaration, the syntax is as follows.
// Assign and initialize the array at the same time as the array declaration
// (*Be careful that the number of array elements to be assigned does not exceed the number of elements of the declared array variable, since it is fixed length)
data type[number of elements] access modifier variable name = array;
Declare the array first and initialize it later
If you only declare the array first and initialize it later, the syntax is as follows.
// Declare only array first
data type[number of elements] access modifier variable name;
// Assign the array to the array variable and initialize it
// (*Be careful that the number of array elements to be assigned does not exceed the number of elements in the declared array variable, since it is of fixed length)
array variable = array;
Variable-Length Array (Dynamic Array)
A Variable-Length Array (Dynamic Array) is “an array that does not have a fixed number of elements (array length)”.
As with Fixed-Length Array, there are two ways to initialize Variable-Length Array.
- Initialize at the same time as the array declaration
- Declare the array first and initialize it later
In the case of Variable-Length Array, it is also possible to initialize the array as a Fixed-Length Array later, after declaring the array.
Initialize at the same time as the array declaration
When initializing an array at the same time as its declaration, the syntax is as follows.
// Assign and initialize the array at the same time as declaring the array
data type[] access modifier variable name = array;
Declare the array first and initialize it later
If you only declare the array first and initialize it later, the syntax is as follows.
// Declare only array first
data type[] access modifier variable name;
// Assign the array to the array variable and initialize it
array variable = array;
To initialize the array as a Fixed-Length Array later, after declaring the array
If the array is declared and later initialized as a Fixed-Length Array, the syntax is as follows.
// Declare only arrays first
data type[] access modifier variable name;
// Initialize as fixed-length array
array variable = new data type[](number of elements);
Sample Code
It is easier to understand if you see the actual code, so a sample code is shown below.
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
/* Fixed-Length Array */
// Initialized at the same time as declaration
uint[3] public initializedFixedArray = [1, 2, 3];
// Declaration only (to be initialized later)
uint[3] public fixedArray;
/* Variable-Length Array (Dynamic Array) */
// Initialized at the same time as declaration
uint[] public initializedDynamicArray = [1, 2, 3, 4, 5];
// Declaration only (to be initialized later)
uint[] public dynamicArray;
// Declaration only (to be initialized later as a fixed-length array)
uint[] public dynamicArray2;
constructor() public {
// Initialization (Fixed-Length Array)
fixedArray = [1, 2, 3];
// Initialization (Variable-Length Array)
dynamicArray = [1, 2, 3, 4, 5];
// Initialized as Fixed-Length Array (variable-length array)
dynamicArray2 = new uint[](3);
}
}
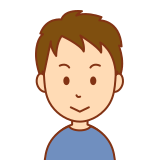
In the sample code, the data type is coded as int, but the method is the same for other data types. Arrays are essential knowledge, so be sure to be able to initialize both Fixed-Length and Variable-Length arrays.