This section explains how to initialize mapping in Solidity.
Syntax
First, declare the mapping as follows.
mapping (key data type => value data type) access qualifier variable name;
Then, initialize the mapping by assigning a value to it.
variable[key] = value;
Sample Code
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
// Declare mapping of "key: address type, value: uint type, access modifier: public"
mapping (address => uint) public balances;
function store(uint256 num) public {
// Initialize mapping with argument num
balances[msg.sender] = num;
}
function retrieve() public view returns (uint256){
return balances[msg.sender];
}
}
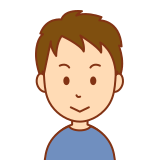
Mappings are what other programming languages call "associative arrays," which are composed of keys and values.