The keccak256 function is, in a word, a "hash function".
However, I don't think it is easy to understand if you are suddenly told that it is a hash function.
This article explains its meaning, results, and usage.
Meaning of hash function
A hash function is "a function that converts a particular value to another value that is irreversible".
The key point is that it is "irreversible (cannot be restored to its original value)".
It is different from encryption because the original value cannot be decrypted from the converted value.
Results of hash function
Let's actually run the keccak256 function and see what value we get.
Specifically, try passing a string to the following code.
keccak256(abi.encodePacked(string))
Passing the value "apple"
Passing the value "apple" returns the hash value "0x85dca312121b082f39dd34192d69dffd93c0add33dac13f7846f2975fba845c6".
No matter how many times this is done, the same result is returned as long as the original value is the same (apple).
Passing the value "appl"
If we pass the value "appl" with only the last character removed, we get "0xc295a848a8ba37d67478c8c0ad603e8ae9b0caa69bf817696bbb7f82d89d78e6d" which is a completely different hash value.
Again, as long as the original value is the same (appl), the same result is returned no matter how many times you do it.
Summary of Results
The results can be summarized as follows.
- Passing a value to the keccak256 function returns a hash value
- Similar characters but one different character will give a completely different hash value
- It is almost impossible to find the original value from the hash value
Usage of hash function
You may have some idea of what hash functions are, but it may be difficult to imagine in what situations they are actually used.
The most typical use of the keccak256 function in Solidity is for string comparisons such as the following.
keccak256(abi.encodePacked(string1)) == keccak256(abi.encodePacked(string2))
In general programming languages, strings can be compared as they are, but Solidity cannot handle strings directly, so it is necessary to convert them to hash values using keccak256 and then compare them.
Note that abi.encodePacked() is a function for handling strings in Solidity.
Sample Code
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
function strToKeccak256(string memory str) public pure returns(bytes32) {
// Hash the string received as argument (str)
return keccak256(abi.encodePacked(str));
}
function isSameWord(string memory word1, string memory word2) public pure returns(bool) {
// Comparing strings (solidity cannot handle strings directly, so hash values are compared to each other)
return keccak256(abi.encodePacked(word1)) == keccak256(abi.encodePacked(word2));
}
}
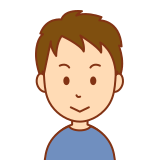
In Solidity, hash values, or keccak256 functions, are often used. Please run the sample code and try entering values to get a feel for the actual behavior.