This section describes the use of the require function in Solidity.
How to use
The require function is used when you want to validate inputs or conditions before executing a process.
It is primarily used to check for errors in external input values or external components.
If the specified condition is not met, processing is aborted and the contract state is restored.
The processed gas cost will be consumed, but the remaining gas cost will be returned.
Syntax
It can simply make a conditional judgment or output an error message.
Conditional only
require(condition)
Also displays error message
require(condition, string)
Display on actual console
If the require condition is not met, you can see that the contract state has been restored.
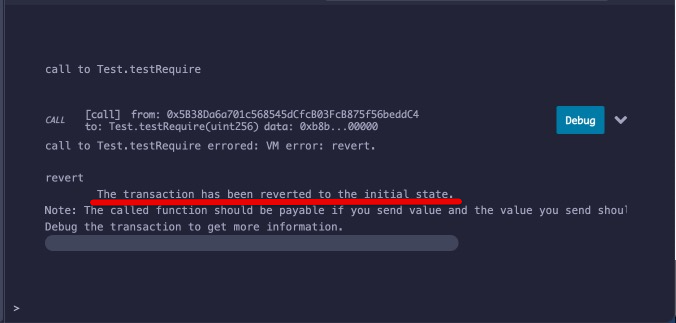
If a string is also passed as an argument, you will see that error messages are also output.
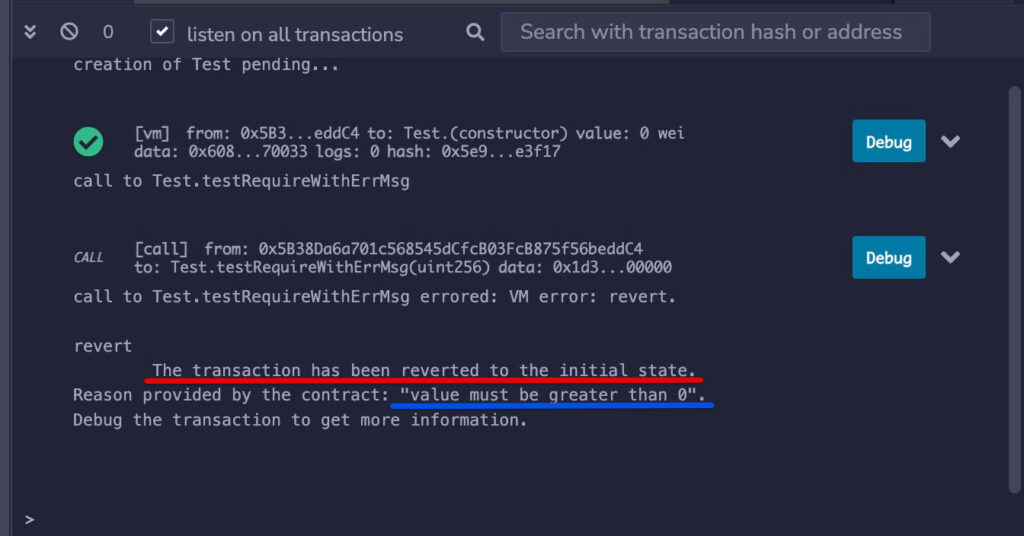
Sample Code
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
function testRequire(uint _value) public pure {
require(_value > 0); // require function (conditional only)
// Process executed when _value is greater than 0
}
function testRequireWithErrMsg(uint _value) public pure {
require(_value > 0, "value must be greater than 0"); // require function (also displays error message)
// Process executed when _value is greater than 0
}
}
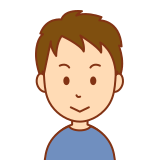
In terms of functions that interrupt processing, it is difficult to distinguish between require/assert/revert, but the require described here is suitable for checking input values. It is also user-friendly, since the remaining gas cost will be returned.