This section describes msg.sender, which is often found in Solidity code.
msg.sender means "caller address"
You can get the caller's account address by writing msg.sender.
Seeing is believing, so let's take a look.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
contract TestContract {
function whoAmI() public view returns(address) {
return msg.sender;
}
}
The value of msg.sender can be obtained by calling the above function.
Looking at the output, we can see that the caller's account address (0x5B38Da6a701c568545dCfcB03FcB875f56beddC4) is displayed.
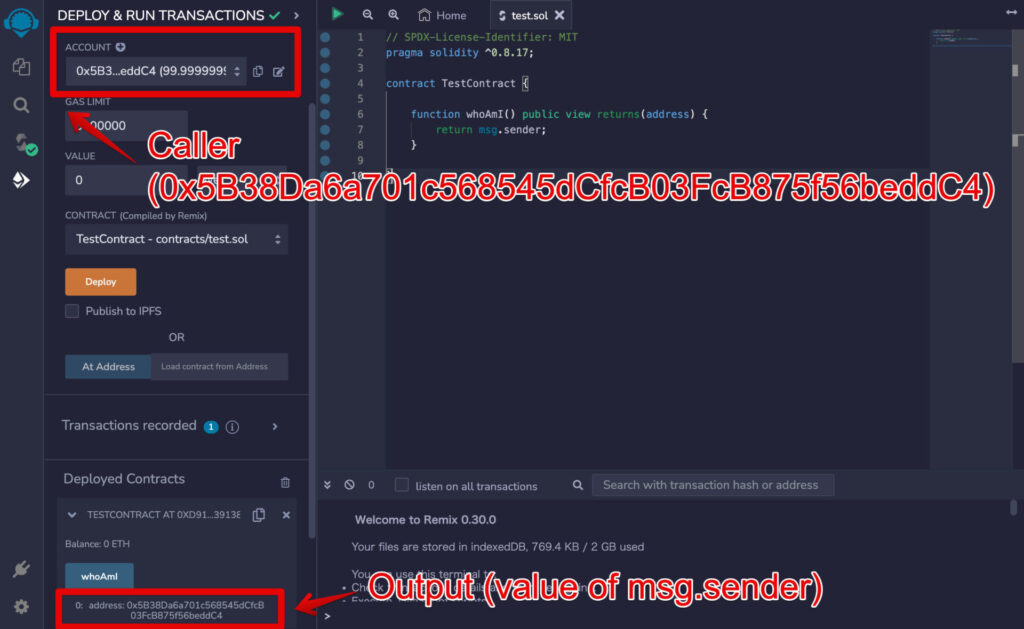
Usage of msg.sender
There are many ways to use msg.sender, but the following two are typical.
- Check if the caller is the owner
- Storing data using the caller's address as a key
Check if the caller is the owner
The sample code below compares the address of the contract owner with the address of the caller and outputs the characters only if they match (only if the caller is the owner).
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
contract TestContract {
address private owner;
constructor() {
owner = msg.sender;
}
function sayHiToOwner() public view returns(string memory) {
require(msg.sender == owner);
return "Hi. Welcome back.";
}
}
Storing data using the caller's address as a key
The sample code below uses the caller's account address as the key to store the data.
This is a common method used to store user-specific data.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
contract TestContract {
mapping (address => string) public userNames;
function setUserName(string memory _userName) public {
userNames[msg.sender] = _userName;
}
function getUserName() public view returns(string memory) {
return userNames[msg.sender];
}
}
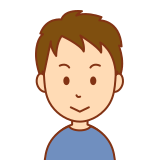
Processing related to contracts such as msg.sender is unique to Solidity, the blockchain development language. It is not so difficult to get used to it, so let's just keep in mind that "msg.sender = caller address".