This section explains how to receive Ether (ETH) in Solidity.
Syntax
To receive Ether (ETH) in Solidity, use the receive or fallback function.
The function modifier "payable" must also be given to make the Ether available for payment.
The following is an explanation of each of these functions.
receive
The receive function is called when msg.data is empty.
You can have one receive function per contract.
receive() access modifier payable {
Processing
}
fallback
The fallback function is called if msg.data has a value or if there is no matching function, including the receive function.
Like the receive function, there can be one fallback function per contract.
fallback() access modifier payable {
Processing
}
Sample Code
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
receive() external payable {
require(msg.value > 0, "Send some ETH to receive.");
}
fallback() external payable {
require(msg.data.length == 0, "Do not call this contract with data");
require(msg.value > 0, "Send some ETH to receive.");
}
function getMyBalance() public view returns(uint) {
return address(this).balance;
}
}
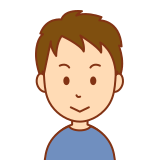
The sample code is only a minimum of the receipt process. In practice, various intermediate processes must be interspersed due to security and tampering countermeasures.