This section explains the basic arithmetic operations (addition/subtraction/multiplication/division) in Solidity.
It also describes remainders and powers.
Syntax
The basic arithmetic operations in Solidity are similar to those in other programming languages.
A table summarizing them is given below.
Calculation | Arithmetic Operator | Example |
---|---|---|
addition | + | a + b |
subtraction | - | a - b |
multiplication | * | a * b |
division | / | a / b |
remainder | % | a % b |
power | ** | a ** b |
Sample Code
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
uint public addition = 1 + 1; // addition
uint public subtraction = 1 - 1; // subtraction
uint public multiplication = 1 * 2; // multiplication
uint public division = 2 / 2; // division
uint public remainder = 3 % 2; // remainder
uint public power = 2 ** 10; // power
}
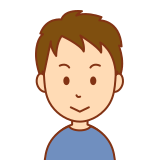
Solidity is unique, but it is a bit of a relief to know that it is the same as a normal programming language when it comes to the basic arithmetic operations.