This section explains how to use the revert function in Solidity.
How to use
The revert function is used when you want to interrupt processing.
When the revert function is reached, processing is interrupted and the contract state is restored.
The processed gas costs will be consumed, but the remaining gas costs will be returned.
Syntax
It can simply suspend processing or output the message.
Process interruption only
revert()
Output the message as well
revert(string)
Display on actual console
You can see that the process is aborted and the contract state is restored.
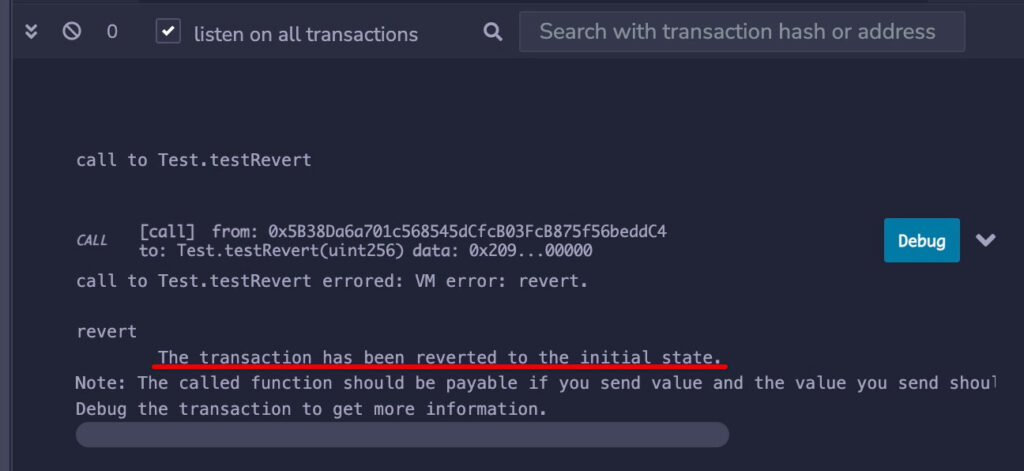
If you pass a string as an argument, you will see that the message is also output.
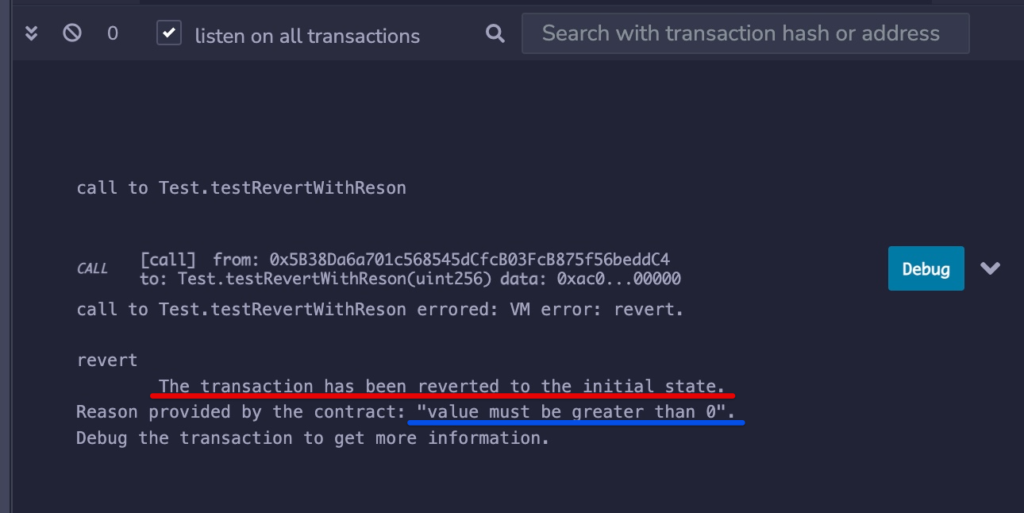
Sample Code
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
function testRevert(uint _value) public pure {
if (_value <= 0) {
revert(); // revert function (process interruption only)
}
// Process executed when _value is greater than 0
}
function testRevertWithReason(uint _value) public pure {
if (_value <= 0) {
revert("value must be greater than 0"); // revert function (output the message as well)
}
// Process executed when _value is greater than 0
}
}
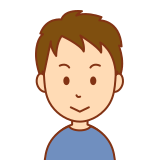
Unlike require and assert, which are functions to suspend processing, revert, described in this article, suspends processing immediately without evaluation. However, it is important to keep it in mind just in case.