This section explains how to write a while/do-while statement in Solidity.
Syntax
To write a while/do-while statement in Solidity, do the following.
while
To write a while statement, do the following.
while(condition) {
Processing
}
do-while
To write a do-while statement, do the following.
Unlike the while statement, execution comes first (the process in the do clause is executed regardless of the loop condition), and then the loop goes around (the process in the do clause is executed) after the loop condition is judged again.
do {
Processing
} while(condition);
Sample Code
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
uint[] numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
function testWhileLoop() public view returns(uint) {
uint sum = 0;
uint8 i = 0;
// while statement (loop as long as the condition is satisfied)
while(i < numbers.length) {
sum += numbers[i];
i++;
}
return sum;
}
function testDoWhileLoop() public view returns(uint) {
uint sum = 0;
uint8 i = 0;
// do-while statement (execution first, then loop as long as condition is satisfied)
do {
sum += numbers[i];
i++;
} while(i < numbers.length);
return sum;
}
}
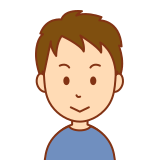
Like the for statement, the use of the while/do-while statement is the same as in other programming languages. It is important to keep them in mind along with arrays.