This section explains the difference between storage and memory, which are handled together with variables in Solidity.
Difference between storage and memory
Both storage/memory are modifiers that indicate where data is to be stored.
storage
Adding the storage modifier to a variable causes its data to be stored on the blockchain.
Variables declared outside of a function (state variables) are treated as storage by default.
Also, a cost (gas cost) is incurred because the data is written to the blockchain.
memory
Adding the memory modifier to a variable causes its data is retained only when the contract is executed.
Function arguments and variables within functions are treated as memory by default.
No cost (gas cost) is incurred because the data is not written to the blockchain.
Summary of storage/memory comparison
The table below compares storage and memory.
Storage Space | Gas Cost | Notes | |
---|---|---|---|
storage | On the blockchain (retained after contract execution) | Yes | Variables declared outside of a function (state variables) are treated as storage by default. |
memory | Memory (discarded after contract execution) | No | Function arguments and variables within functions are treated as memory by default. |
Sample Code
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
// Variables declared outside of a function (state variables)
// are treated as storage by default.
string str = "hello";
// Function arguments and variables within functions
// are treated as memory by default.
function multiple(uint x) public pure returns(uint) {
uint y = 10;
return x * y;
}
}
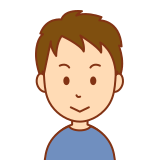
Since the use of storage incurs costs (gas costs), code should be written with an eye to avoiding the use of storage as much as possible. In Solidity, in addition to implementation, it is also necessary to be conscious of keeping costs down.