This section provides a summary of data types in Solidity.
List of Solidity data types
The table below lists the Solidity data types.
Data Type | Possible Value | Default Value | Remarks |
---|---|---|---|
bool | Boolean (true/false) | false | - |
int | Signed integer (-2 ** n-1 to 2 ** n-1 - 1) | 0 | Exists from int8 to int256 and 8 bits each. int is alias with int256. |
uint | Unsigned integer (0 to 2 ** n - 1) | 0 | Exists from uint8 to uint256 and 8 bits each. uint is alias with uint256. |
fixed | Signed fixed-point number | 0 | Exists from fixed8 to fixed256 and 8 bits each. fixed is alias with fixed256. |
ufixed | Unsigned fixed-point number | 0 | Exists from ufixed8 to ufixed256 and 8 bits each. ufixed is alias with ufixed256. |
address | Ethereum Address | address(0)(0x0000000000000000000000000000000000000000) | - |
bytes | Byte array | 0x | - |
string | String | "" (empty string) | - |
enum | Enumerative type | First element in an enumerated type. | Enumerate constants (values are assigned sequentially with the first element as 0). |
struct | Structure | - | Combine variables of different data types into a single type. |
mapping | Mapping - Key/Value Pairs | Depends on the data type of the mapping value. | - |
array | Array | [] (empty array) | Two types of arrays exist: fixed-length and variable-length. |
Sample Code
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
/* bool (boolean) */
bool varBool = true;
/* int (signed integer) */
int varInt = -1;
int varInt8 = 1;
int varInt16 = -1;
int varInt32 = 1;
int varInt64 = -1;
int varInt128 = 1;
/* uint (unsigned integer) */
uint varUint = 1;
uint varUint8 = 1;
uint varUint16 = 1;
uint varUint32 = 1;
uint varUint64 = 1;
uint varUint128 = 1;
/* fixed (signed fixed-point number) */
fixed varFixed = -0.1;
fixed varFixed8 = 0.1;
fixed varFixed16 = -0.1;
fixed varFixed32 = 0.1;
fixed varFixed64 = -0.1;
fixed varFixed128 = 0.1;
/* ufixed (unsigned fixed-point number) */
ufixed varUfixed = 0.1;
ufixed varUfixed8 = 0.1;
ufixed varUfixed16 = 0.1;
ufixed varUfixed32 = 0.1;
ufixed varUfixed64 = 0.1;
ufixed varUfixed128 = 0.1;
/* address (Ethereum address) */
address varAddress = 0x0000000000000000000000000000000000000000;
/* bytes (byte array) */
bytes varBytes = "0x0";
bytes1 varBytes1 = "0";
bytes32 varBytes32 = "0x1234567890";
/* string */
string varString = "hello";
/* enum (enumerated type) */
enum Colors {
Red, // 0
Green, // 1
blue // 2
}
/* struct (structure) */
struct User {
uint id;
string name;
address balance;
}
/* mapping (mapping - key/value pairs) */
mapping (address => uint) balances;
/* array */
// fixed-length array
string[2] members = ["bob", "alice"];
// variable-length array
uint[] numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
}
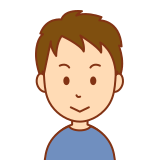
Each data type has a different cost (gas cost). When creating more sophisticated applications, it is important to understand the cost of each data type.