This section explains how to write logical operators (and/or) in Solidity.
Syntax
The following is how to write logical operators (and/or) in Solidity.
And (logical conjunction)
"And (logical conjunction)" is written with && followed by two ampersands (&).
condition 1 && condition 2
Or (logical disjunction)
"Or (logical disjunction)" is written with || followed by two vertical bars (|).
condition 1 || condition 2
To combine multiple conditions
To group multiple conditions together, enclose the clusters of conditions in parentheses (()).
(condition 1 && condition 2) && (condition 3 || condition 4)
Sample Code
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
function sum(uint x, uint y) public pure returns(uint) {
uint sum;
// x > 0 and y > 0
if (x > 0 && y > 0) {
// x >= 100 or y >= 100
if (x >= 100 || y >= 100) { revert("values must be less than 100"); }
sum = x + y;
}
return sum;
}
}
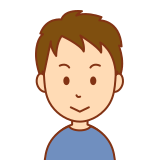
Solidity has a unique syntax, but the logical operators are the same as in many other programming languages!