This section describes "onlyOwner", which is always found in Solidity code.
What is onlyOwner?
onlyOwner is "a modifier that makes it callable only by its contract owner".
By modifying a function with onlyOwner, the function can be restricted so that only the owner of the contract can call it. (*Please see the following related article for more information on modifiers)
Contents of onlyOwner
onlyOwner is used to qualify the function and must be predefined.
In most cases, the implementation will look something like the following. (Conversely, if the contents of onlyOwner are messed up, the processing of onlyOwner itself will also change.)
address owner;
constructor() {
owner = msg.sender;
}
modifier onlyOwner {
require (msg.sender == owner);
_;
}
The "require (msg.sender == owner)" in the contents of onlyOwner is the key.
It checks if the caller is the owner, and if not, aborts the process.
As for the "_;", it means "continue processing" and is used to ensure that the function qualified by onlyOwner continues to execute as is. (Without this description, even if the function is qualified with onlyOwner, the processing of the function will not continue and will stop at onlyOwner.)
On the other hand, when onlyOwner is qualified to a function, it becomes as follows.
function sayHi() public onlyOwner view returns(string memory) {
return "Hi Owner!";
}
Simply appending onlyOwner to a function in this way allows the process defined by onlyOwner (= only its contract owner can call it) to be executed before the function is called.
In other words, the sayHi function above can only be called by the owner of its contract.
Sample Code
The full sample code, including the definition of onlyOwner and modifications to the function, is shown below.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
contract Sample {
// Define onlyOwner modifier
modifier onlyOwner {
require (msg.sender == owner); // if the caller is not the owner, the process is aborted
_; // continue processing the modified function
}
// Modifying the function with the onlyOwner modifier (so that it can only be called by the contract owner)
function sayHi() public onlyOwner view returns(string memory) {
return "Hi Owner!";
}
}
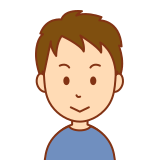
Although the modifier named "onlyOwner" appears frequently and is therefore explained specifically for onlyOwner, onlyOwner is only one of the modifier modifiers. By creating your own modifier modifiers, you can use them in the same way as onlyOwner, so it is important to master the modifier modifiers.