This section explains how to write the enum (enumerated type) in Solidity.
Syntax
Here is how to write the enum (enumerated type) in Solidity.
The first constant is set to 0, and the values are assigned in order from 0.
enum variable name {
constant 1,
constant 2,
constant n
}
Sample Code
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
// Enumerated Type
enum Colors {
None, // 0
Red, // 1
Green, // 2
Blue // 3
}
Colors color = Colors.None;
function setRed() public {
color = Colors.Red;
}
function setGreen() public {
color = Colors.Green;
}
function setBlue() public {
color = Colors.Blue;
}
function clearColor() public {
color = Colors.None;
}
function getColor() public view returns(Colors) {
return color;
}
}
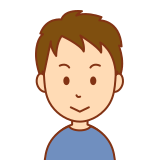
Enum (enumerated type) is useful when you want to group certain constants together. It also improves the outlook of the code, so it should be used proactively.