This section explains how to output the debug log in Solidity.
It is assumed that you are using Remix (Ethereum IDE).
Syntax
This section describes how to output and check the debug log.
How to output
To output the debug log in Solidity, do the following
The description of event/emit is a set.
You can also set multiple arguments to an event.
event event name(argument 1[data type], argument 2[data type], ..., argument n[data type]);
emit event name(value 1, value 2, ..., value N);
How to confirmation
To view the debug log in Solidity, expand the arrow icon to the right of the "Debug" button.
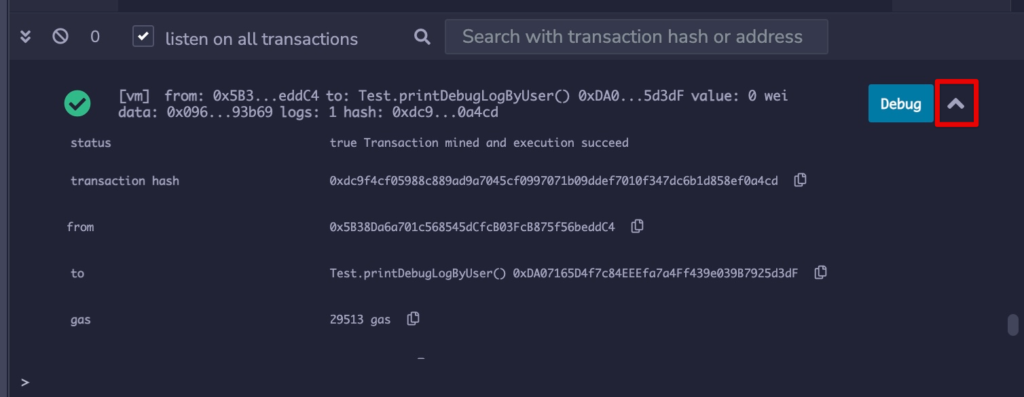
Then, check the values of "event" and "args" by looking at the "logs" entry in the debug contents.
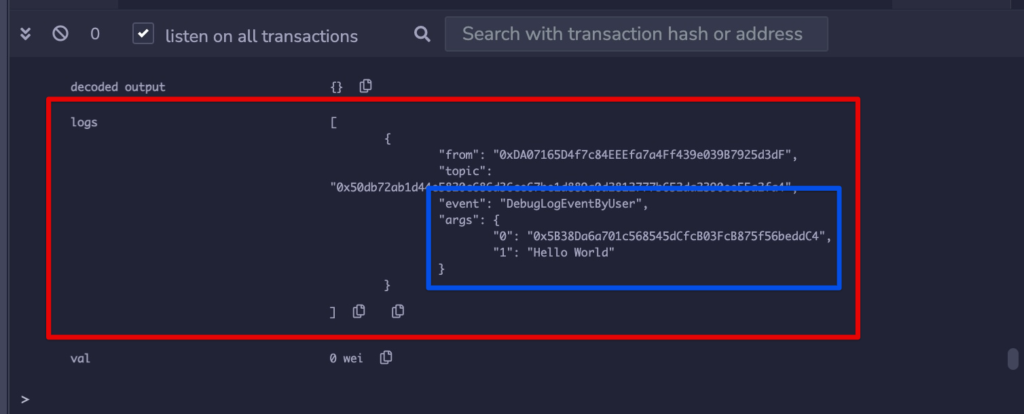
Sample Code
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
event DebugLogEvent(string); // single argument
event DebugLogEventByUser(address, string); // multiple argument
string message = "Hello World";
function printDebugLog() public {
emit DebugLogEvent(message);
}
function printDebugLogByUser() public {
emit DebugLogEventByUser(msg.sender, message);
}
}
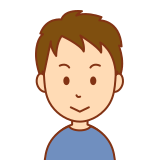
Solidity logging is a bit confusing. However, log output is essential for writing code, so let's master it firmly.