This section explains how to write a comment (comment-out) in Solidity.
Syntax
The way to write a comment in Solidity is basically the same as in other programming languages.
However, there is a NatSpec (Solidity's own format), which is also described here.
One-line Comment
A double slash (//) is followed by a comment.
// This is a one-line comment
// This is a one-line comment
// This is a one-line comment
Multi-line Comment
Enclose comments with slashes and asterisks (/* - */).
/*
This is a multi-line comment
This is a multi-line comment
This is a multi-line comment
*/
NatSpec (solidity's own format)
Solidity has its own format for comments to developers and users.
It is called NatSpec (Ethereum Natural Language Specification Format).
The comment-out format is slightly different from the usual format, and has its own tags.
Comment-out
In NatSpec, one-line comments are enclosed with triple slashes (///), and multi-line comments are enclosed with slashes and double asterisks to slashes and asterisks (/** - */).
/// @title title
/// @author author
/**
* @notice Description for users
* @dev Detailed description for developers
*/
Tags
The table below lists the tags in NatSpec.
Tag | Description | Context |
---|---|---|
@title | Contract/Interface title | contract, library, interface |
@author | Author name | contract, library, interface |
@notice | Description of what it does for users | contract, library, interface, function, public state variable, event |
@dev | A more detailed description of what it does for developers | contract, library, interface, function, state variable, event |
@param | Parameter description | function, event |
@return | Return value description | function, public state variable |
@inheritdoc | Copy all missing tags in the base function | function, public state variable |
@custom:... | Custom tag | everywhere |
Sample Code
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
/// @title Test Contract
/// @author Sloth
/// @notice Contract for testing
/// @dev Contract for various development tests
/// @custom:test This is a test contract.
contract Test {
/**
* @notice Calculating addition
* @dev Addition of unsigned integer values
* @param {uint} x
* @param {uint} y
* @return {uint} sum total
*/
function add(uint x, uint y) public pure returns(uint) {
return x + y;
}
}
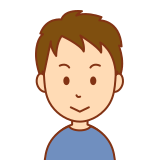
Be sure to write comments frequently. It will help you modify your code later, and it will also make it easier for other developers to understand the process.