This section describes Solidity's access modifiers.
There are four types of access modifiers: public/private/internal/external.
public
If the public modifier is added, it can be accessed directly from the outside.
It can also be called from within the contract or from an inherited contract.
The execution cost (gas cost) of a function call is higher than that of an external modifier because the argument values are stored in memory.
data type public variable name;
function function name (data type argument) public [function modifier] [returns(data type)] {
}
private
If the private modifier is added, it can be accessed only from within the same smart contract.
Also, it cannot be called from an inherited contract.
data type private variable name;
function function name (data type argument) private [function modifier] [returns(data type)] {
}
internal
If the internal modifier is added, it can be accessed only from within the same smart contract.
However, unlike the private modifier, it can be called from an inherited contract.
data type internal variable name;
function function name (data type argument) internal [function modifier] [returns(data type)] {
}
external
If the external modifier is added, it can be accessed only from the external smart contract.
Also, unlike the public modifier, it cannot be called from within the contract or from an inherited contract.
In a function call, the argument values are not stored in memory, so the execution cost (gas cost) is lower than with the public modifier.
function function name (data type argument) external [function modifier] [returns(data type)] {
}
Comparative Summary of Access Modifiers
The following table summarizes the comparison of each access modifier.
Contract Internal | External Contract | Inherited Contract | Target (variable/function) | Remarks | |
---|---|---|---|---|---|
public | ○ | ○ | ○ | both | Higher cost (gas cost) compared to external |
private | ○ | × | × | both | - |
internal | ○ | × | ○ | both | - |
external | × | ○ | × | function only | Lower cost (gas cost) compared to public |
Sample Code
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
/* variable */
// Public
uint256 public publicVariable = 10;
// Private
uint256 private privateVariable = 10;
// Internal
uint256 internal internalVariable = 10;
/* function */
// Public
function publicFunction(uint num) public pure returns(uint) {
return num;
}
// Private
function privateFunction(uint num) private pure returns(uint) {
return num;
}
// Internal
function internalFunction(uint num) internal pure returns(uint) {
return num;
}
// External
function externalFunction(uint num) external pure returns(uint) {
return num;
}
}
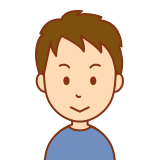
The most important thing to keep in mind when writing smart contracts is security. One wrong move can lead to a situation where all the funds stored on the smart contract can be taken away. For this reason, make sure you master the characteristics of each access modifier.