This section explains how to use the break/continue statement.
Syntax
The usage of the break/continue statement is as follows.
break
By inserting a break statement inside the loop, the loop itself is broken when the break is reached.
A break statement is written as follows.
break;
continue
By inserting a continue statement in the loop, the program will skip further processing when it reaches the continue point.
Unlike the break statement, it does not exit the loop itself, but only skips processing after the continue statement. (The loop continues and processing before the continue statement is performed).
A continue statement is written as follows.
continue;
Sample Code
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
uint[] numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
function testBreak() public view returns(uint) {
uint sum = 0;
uint8 i = 0;
while(i < numbers.length) {
sum += numbers[i];
i++;
// If sum is 6 or more, exit the loop itself (the while statement itself)
if (sum >= 6) { break; }
sum += 1;
}
return sum;
}
function testContinue() public view returns(uint) {
uint sum = 0;
uint8 i = 0;
while(i < numbers.length) {
sum += numbers[i];
i++;
// If sum is 6 or greater, skip subsequent processing (lines 34-35)
if (sum >= 6) { continue; }
sum += 1;
}
return sum;
}
}
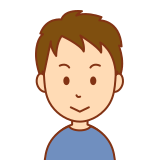
The break/continue statement is necessary for more flexible loop processing, and should be kept in mind along with the for and while statements.