This section describes how to check for the existence of a mapping in Solidity.
Conclusion: Mapping has no method to check for existence
First of all, to conclude, Solidity's mapping does not provide a method to check for the existence of a value, such as exists method.
Therefore, it is necessary to take alternative measures, such as checking for the existence of a value by comparing it with an initial value.
Alternative: Compare with the initial value and check for existence
In Solidity mapping, if a key does not exist, it will not be empty (null) but will return an initial value according to its type.
This property is used to check for the existence of a mapping.
Datatype | Default Value |
---|---|
uint, int | 0 |
bool | false |
address | address(0)(0x0000000000000000000000000000000000000000) |
bytes | 0x |
string | "" (empty string) |
Since the table above is the initial value for each data type, it is compared with this to check for existence.
The following is an actual sample code.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
contract TestContract {
mapping (address => uint) private uintMapping; // uint
mapping (address => bool) private boolMapping; // bool
mapping (address => address) private addressMapping; // address
mapping (address => bytes) private bytesMapping; // bytes
mapping (address => string) private stringMapping; // string
function isExistUint() public view returns(string memory) {
if (uintMapping[msg.sender] == 0) {
return "Not Exitst";
}
return "Exist";
}
function isExistBool() public view returns(string memory) {
if (boolMapping[msg.sender] == false) {
return "Not Exitst";
}
return "Exist";
}
function isExistAddress() public view returns(string memory) {
if (addressMapping[msg.sender] == address(0)) {
return "Not Exitst";
}
return "Exist";
}
function isExistBytes() public view returns(string memory) {
if (keccak256(bytesMapping[msg.sender]) == keccak256(hex"")) {
return "Not Exitst";
}
return "Exist";
}
function isExistString() public view returns(string memory) {
if (keccak256(abi.encodePacked(uintMapping[msg.sender])) == keccak256(abi.encodePacked(""))) {
return "Not Exitst";
}
return "Exist";
}
}
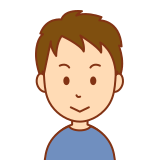
This is not a strict method, but we have introduced it as an alternative. If Solidity's built-in methods (standard methods) are not available, you should create your own or use an external library.