This section explains how to write comparison operators (equal/not equal) in Solidity.
Syntax
The following is how to write comparison operators (equal/not equal) in Solidity.
Equal (equivalent)
"Equal (equivalent)" is written with == followed by two equals (=).
value 1 == value 2
Not Equal (inequivalent)
"Not equal (inequivalent)" is indicated by ! = and exclamation mark (!) and an equal (=) is written.
value 1 != value 2
To combine multiple conditions
To group multiple conditions together, enclose the clusters of conditions in parentheses (()).
(value 1 == value 2) && (value 3 != value 4)
Sample Code
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
function evenOrOdd(uint num) public pure returns(string memory) {
string memory message = "";
// The remainder of 2 dividing num is zero
if (num % 2 == 0) {
message = "even";
}
// The remainder of 2 dividing num is not zero
if (num % 2 != 0) {
message = "odd";
}
return message;
}
}
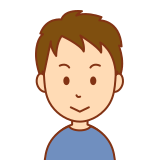
Solidity has a unique syntax, but the comparison operators are the same as in many other programming languages!