This section describes state variables in Solidity.
What is a state variable anyway?
A state variable in Solidity is a "variable stored in a contract".
Since it is stored in the contract, the data is retained even after the contract is executed.
Conversely, memory variables, which are the counterpart of state variables, are destroyed after contract execution.
Variables declared outside of a function are state variables
Variables declared outside of a function are by default state variables.
This means that if you declare a variable outside of a function, it will be permanently stored in the contract.
State variables have access levels
State variables have access levels (scope of variable disclosure).
The following access levels are available.
- public: Can be accessed directly from the outside.
- private: Accessible only from within the same smart contract (not from an inherited party).
- internal: Accessible only from within the same smart contract (or from an inherited party).
Sample Code
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
/* State variables (variables declared outside of a function are state variables by default) */
// Public
uint256 public publicVariable = 10;
// Private
uint256 private privateVariable = 10;
// Internal
uint256 internal internalVariable = 10;
function sampleFunction(uint num) public pure returns(uint) {
return num;
}
}
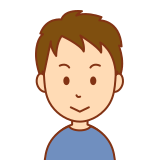
Although we usually use variables without thinking about them, it is important to remember that there are "state variables" and "memory variables". The former are variables that remain stored in the contract (blockchain) after the contract is executed, while the latter are variables that are destroyed after the contract is executed. Variables declared outside of a function become state variables, while those declared within a function become memory variables.