This section explains how to get the mapping length in Solidity.
Conclusion: There is no method to get the length of a mapping
Solidity's mapping does not provide methods for obtaining lengths, such as length method.
Therefore, you will need to take alternative measures, such as preparing a dedicated variable to store the length.
Alternative: Provide a variable to manage length
The following is sample code with variable to manage the length of the mapping.
It is incremented (number plus 1) each time an element is added to the mapping and decremented (number minus 1) each time an element is removed.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
contract TestContract {
mapping (address => string) public users;
uint userNum = 0; // variable that manages the length of the users mapping
function addUser(string memory _userName) public {
users[msg.sender] = _userName;
userNum++; // increment each time an element is added
}
function delUser(address _userAccount) public {
users[_userAccount];
userNum--; // decrement each time an element is deleted
}
// Get the number of elements in the users mapping
function getUserNum() public view returns(uint) {
return userNum;
}
}
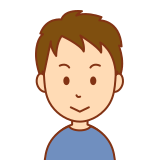
Because Solidity is a blockchain development language, its available methods are more limited than those of other programming languages. Therefore, for operations that are not available in the methods, implement your own processing or use external libraries.