This section describes how to write a function in Solidity.
Syntax
The syntax of the function is as follows.
There are two types of functions: "without return value (only processing is performed)" and "with return value (processing result is also returned)".
Without return value (only processing is performed)
If there is no return value (only processing is performed), the function is written as follows.
function function name ([data type argument]) [access modifier] [state modifier] {
// Processing
}
With return value (processing result is also returned)
If there is a return value (processing result is also returned), the function is written as follows.
function function name ([data type argument]) [access modifier] [state modifier] returns(data type) {
// Processing
return value (same data type as specified for returns);
}
Syntax Details
The following is a detailed description of the syntax.
function name
MixedCase (initial letters capitalized except for the first word) is recommended for function names.
It is also recommended that private functions be prefixed with an underscore (_).
data type
The main data types are as follows.
- bool:Boolean
- int:Signed integer
- uint:Unsigned integer
- fixed:Signed fixed-point number
- ufixed:Unsigned fixed-point number
- address:Ethereum Address
- bytes:Byte array
- string:String
- enum:Enumerative type
- struct:Structure
- mapping:Mapping - Key/Value Pairs
- array:Array
For more information, please click here.
access modifier
The main access modifiers are as follows.
- public: it can be accessed directly from the outside / it can also be called from within the contract or from an inherited contract
- private: it can be accessed only from within the same smart contract / it cannot be called from an inherited contract
- internal: it can be accessed only from within the same smart contract / it can be called from an inherited contract
- external: it can be accessed only from the external smart contract / it cannot be called from within the contract or from an inherited contract
For more information, please click here.
state modifier
The main state modifiers are as follows.
- view: State variable (storage variable) is not changed / Readable
- pure: Do not read/change state variables (storage variables) / Arguments can be read/changed
- payable: Possible to receive eth (Ethereum)
Sample Code
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
contract TestContract {
string greeting = "hi";
function sayHi() public view returns(string memory) {
return greeting;
}
function _sayHi() private view returns(string memory) {
return greeting;
}
function sum(uint x, uint y) public pure returns(uint) {
return x + y;
}
function _sum(uint x, uint y) private pure returns(uint) {
return x + y;
}
}
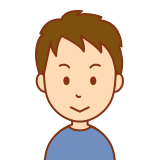
The basic syntax is similar to other programming languages, but only the function modifiers (access modifiers and state modifiers) may be unique to Solidity. Be sure to keep each of these characteristics in mind.