This section explains how to use the switch statement in Solidity.
Conclusion: The switch statement is not supported in Solidity
The current version of Solidity (v0.8.x) does not support the switch statement.
Therefore, you need to use the if-elseif statement instead, or take other alternative measures.
Alternative: Use the if-elseif statement
Since the switch statement cannot be used, the if-elseif statement is substituted.
Suppose you want to write the following switch statement in another language (Javascript).
function getColor(selected) {
switch (selected) {
case 0:
return "red";
case 1:
return "green";
case 2:
return "blue";
default:
return "white";
}
}
In that case, rewriting the if-elseif statement in Solidity would look like this.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
contract Sample {
function getColor(uint selected) public pure returns (string memory) {
if (selected == 0) {
return "red";
} else if (selected == 1) {
return "green";
} else if (selected == 2) {
return "blue";
} else {
return "white";
}
}
}
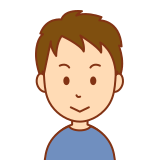
Solidity often does not allow the use of grammars supported by other languages. In such cases, take alternative measures, such as using a different grammar.