This section describes "constructor", which are always found in Solidity code.
What is a constructor anyway?
A constructor is used to initialize the state of the contract.
The code in the constructor function is executed only once when the contract is deployed.
Therefore, initial values, etc. that you want to set in advance in the contract should be written in the constructor.
How to write a constructor?
A constructor is written as follows.
You can also pass arguments to the constructor function.
contract MyContract {
// constructor
constructor([arguments]) {
Processing
}
}
An actual example is shown below.
If you want to see not only the constructor part but also the full code, please refer to "Sample Code" in the next chapter.
contract TestContract {
address private owner;
uint private balance;
constructor() {
owner = msg.sender;
balance = 1000;
}
}
Sample Code
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
contract TestContract {
address private owner;
uint private balance;
constructor() {
owner = msg.sender;
balance = 1000;
}
function isOwner() public view returns(bool) {
return msg.sender == owner;
}
function getBalance() public view returns(uint) {
return balance;
}
}
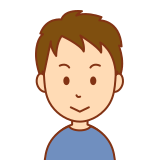
You must know how to use constructors when writing Solidity code. Make sure you master it.