This section describes OpenZeppelin, which is always used for Solidity development.
Knowing OpenZeppelin will greatly expand the scope of your development.
OpenZeppelin is a framework for smart contract development
OpenZeppelin is, in a nutshell, a "smart contract development framework".
It is written in Solidity, a blockchain development language.
By using OpenZeppelin, complex processes in Solidity development can be written in simple code, and development can be done much more smoothly than with Solidity alone.
The following is the official OpenZeppelin documentation.
OpenZeppelin Usage
To use OpenZeppelin, import OpenZeppelin into your current code.
A sample code is shown below.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
// Import OpenZeppelin Math library
import "@openzeppelin/contracts/utils/math/Math.sol";
contract TestContract {
function getMax(uint a, uint b) public pure returns(uint) {
// Use max method of Math library
return Math.max(a, b);
}
}
Above we import the Math library as an example, but there are many others.
The list of libraries can be found in the "API" section of the Contracts page.
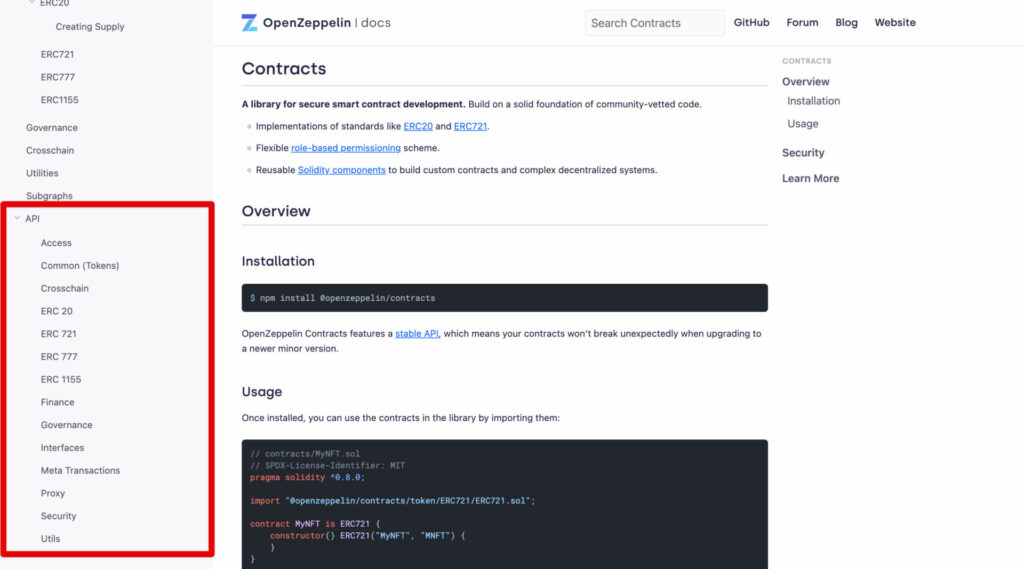
OpenZeppelin Usage Example
Some examples of OpenZeppelin use are shown below.
Access Control
The following is sample code that uses the onlyOwner modifier of an Ownable contract to prohibit execution by anyone other than the contract owner.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
// Import Ownable contract in OpenZeppelin
import "@openzeppelin/contracts/access/Ownable.sol";
contract TestContract is Ownable {
// Qualified by onlyOwner of Ownable contract
function sayHelloToOwner() public onlyOwner view returns(string memory) {
return "Hi Owner";
}
}
Tokens ERC20
The following is sample code that uses the _mint method of the ERC20 contract to issue a unique token called Sloth (SLTH).
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
// Import ERC20 contract in OpenZeppelin
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
contract SLTHToken is ERC20 {
constructor(uint256 initialSupply) ERC20("Sloth", "SLTH") {
// Use _mint method of ERC20 contract
_mint(msg.sender, initialSupply);
}
}
Utilities
The following is sample code that defines AddressToUintMap using the EnumerableMap utility library.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.17;
// Import EnumerableMap library in OpenZeppelin
import "@openzeppelin/contracts/utils/structs/EnumerableMap.sol";
contract Test {
// Import AddressToUintMap from library
using EnumerableMap for EnumerableMap.AddressToUintMap;
// Define AddressToUintMap
EnumerableMap.AddressToUintMap private myTestMap;
function setValue(uint num) public {
// Use set method of EnumerableMap
EnumerableMap.set(myTestMap, msg.sender, num);
}
function getValue() public view returns(uint) {
// Use get method of EnumerableMap
return EnumerableMap.get(myTestMap, msg.sender);
}
function existValue() public view returns(bool) {
// Use contains method of EnumerableMap
return EnumerableMap.contains(myTestMap, msg.sender);
}
function countValue() public view returns(uint) {
// Use length method of EnumerableMap
return EnumerableMap.length(myTestMap);
}
}
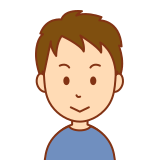
By using OpenZeppelin, you can write very simple code for processes that would be very complex and long if written in Solidity alone. It is often used to issue tokens and mint NFTs, so it is important to master it.