This section explains how to get the current date and time in Solidity.
Syntax
To get the current date and time in Solidity, use block.timestamp.
The current date and time is returned as a UNIX timestamp.
block.timestamp
Time Units are also available, as shown in the table below.
suffix | unit | number of seconds |
---|---|---|
weeks | week | 60 * 60 * 24 * 7 |
days | day | 60 * 60 * 24 |
hours | hour | 60 * 60 |
minutes | minute | 60 |
seconds | second | 1 |
Suffixes are written in the form of "number + suffix" as shown here.
Of course, addition and other four arithmetic operations are also possible.
n weeks; n days; n hours; n minutes; n seconds;
Sample Code
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
function getCurrentTimestamp() public view returns(uint) {
// Get the current date and time (timestamp)
return block.timestamp;
}
function getTotalTime() public pure returns(uint) {
// Add each time unit (seconds)
return 1 weeks + 1 days + 1 hours + 1 minutes + 1 seconds;
}
function hasExpired(uint timestamp) public view returns(bool) {
// Whether a day has passed
return block.timestamp > timestamp + 1 days;
}
}
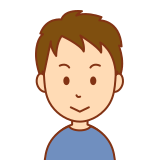
Please note that the now function, which was used frequently in the past, has been deprecated and is no longer available.