This section explains how to delete mapping element in Solidity.
Syntax
To delete a mapping element in Solidity, use the delete operator.
delete Mapping Variable[key];
Sample Code
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
mapping (address => uint) public balances;
function store(uint256 num) public {
balances[msg.sender] = num;
}
function deleteElement(address _address) public {
// Delete mapping element
delete balances[_address];
}
function retrieve() public view returns (uint256){
return balances[msg.sender];
}
}
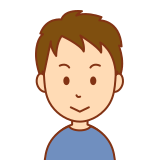
Deleting an element with the delete operator will only result in 0 being stored. Note that the element itself is not deleted, and the number of elements itself remains unchanged.