The following is a summary of the default values (initial values) for mapping in Solidity.
The default values for mappings depend on the data type of the values set for the mapping.
They are explained in detail below.
Default value for mapping per value data type
The “data type of the value” refers to the bold marker here.
mapping (key datatype => value datatype) access qualifier variable name;
As mentioned at the beginning of this text, the default values for mapping are different for each value data type.
The following is a list of them.
Value Datatype | Default Value |
---|---|
uint, int | 0 |
bool | false |
address | address(0)(0x0000000000000000000000000000000000000000) |
bytes | 0x |
string | ""(empty string) |
Sample Code
I have created a function that returns a default value for each data type.
Please run and check it.
// SPDX-License-Identifier: GPL-3.0
pragma solidity >=0.7.0 <0.9.0;
contract Test {
mapping (address => uint) public uintMapping;
mapping (address => int) public intMapping;
mapping (address => bool) public boolMapping;
mapping (address => address) public addressMapping;
mapping (address => bytes) public bytesMapping;
mapping (address => string) public stringMapping;
function getDefaultValueUint() public view returns (uint) {
return uintMapping[msg.sender];
}
function getDefaultValueInt() public view returns (int) {
return intMapping[msg.sender];
}
function getDefaultValueBool() public view returns (bool) {
return boolMapping[msg.sender];
}
function getDefaultValueAddress() public view returns (address) {
return addressMapping[msg.sender];
}
function getDefaultValueBytes() public view returns (bytes memory) {
return bytesMapping[msg.sender];
}
function getDefaultValueString() public view returns (string memory) {
return stringMapping[msg.sender];
}
}
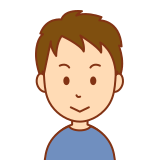
Since these initial values are sometimes used to check for the presence of keys in mappings, it is important to keep the default values for each data type in mind.